Override components
Provide a means of extending the Formatic
library by allowing you to render out your own, custom React
components in place of native library components.
Overview
Whilst we try to make all of our components highly customizable through our cloud dashboard, we recognize that there will be times where developers will want to make changes to the component itself. One such example might be implementing a custom JS library on all Text
field components, which in part requires a change to the DOM structure. This would essentially mean implementing a custom render()
method on the Text
component. Rather than forking our library and customizing the component, we simply allow you to pass through your own, custom component which Formatic
will use to render out Text
fields in its place.
Formatic
exports an Overrides
object, containing a set of components used to override different types of components within the library. To import:
import Formatic, { Overrides } from '@formatic/sdk';
The Overrides
object contains the following components:
Overrides.OverrideField
- Override a field by ID or type.
To override components, simply pass through as children components of the main Formatic
component. See below for examples.
Note
Further release candidates will export additional overrides, including Section, Page and System components.
OverrideField
Used to override field components by ID or type. Contains the following props:
component
Object Component to pass through as an override.type
String The type of component to override, e.g.text
. Optional.id
String The ID of the field to override. Optional.
Fields are the most common form of overrides. A basic form without customization may look like:
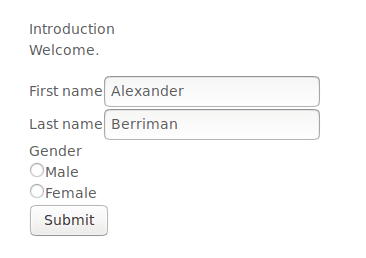
Creating your override
Before overriding, you first need to create your own custom component that you want to use in place of a native Formatic
component. A simple example may be:
import React, { Component } from 'react';
export default class CustomTextComponent extends Component {
render() {
return (
<div className="custom-component">
<h3>My Custom Text Component</h3>
<input type="text" placeholder="Custom component" />
</div>
)
}
}
By field type
If you then want to use your component to override all fields by type, pass through the type
prop.
import React, { Component } from 'react';
import Formatic, { Overrides } from '@formatic/sdk';
import CustomTextComponent from './components/CustomTextComponent';
class App extends Component {
render() {
return (
<Formatic {...props}>
<Overrides.OverrideField component={CustomTextComponent} type="text" />
</Formatic>
);
}
}
This will render out a form as follows:
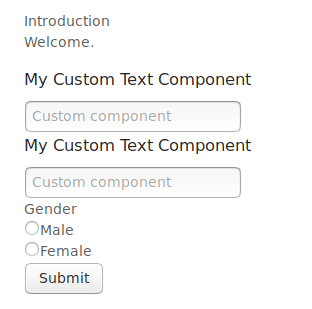
As you can see, all Text
fields are now rendered using your CustomTextComponent
.
By field ID
Optionally, you can override a field by ID. This is useful when you want to override a specific field, rather than all of them. To override by ID, pass the ID of the field through the id
prop:
import React, { Component } from 'react';
import Formatic, { Overrides } from '@formatic/sdk';
import CustomTextComponent from './components/CustomTextComponent';
class App extends Component {
render() {
return (
<Formatic {...props}>
<Overrides.OverrideField component={CustomTextComponent} id="FIELD_ID" />
</Formatic>
);
}
}
This will render out a form as follows:
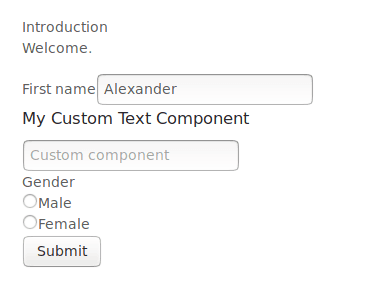
Where the Last Name
form field has been overridden.